LAB 2 – INSTRUCTIONS
OVERVIEW
THIS IS AN EXTENSION OF LAB 1. NEW PARTS ARE HIGHLIGHTED.
Again, it should be emphasized that this exercises are not about teaching “web design” or “web programming” in general; instead we are just using the browser as a “platform” for our actual scripts… and that the creation and execution of these scripts requires no tools above and beyond what you likely already have installed on any machine… a web browser and a text editor.
OBJECTIVE
Create a Guess The Number (Higher / Lower) Game, based on the instructions below, where the computer tries to guess the number YOU are thinking. Note: the “Design” is up to you as long as it meets the minimal requirements as stated in the instructions.
INSTRUCTIONS
- A “game set-up” area that is displayed first.
- Before displaying the main “game” area:
- Show 2 “input” fields on the screen where the user the user can tell the computer the “range” of numbers to choose from. For Example:
I am thinking of a number between and . - An “input” field where the user can choose the maximum number of computer guesses. For Example:
Maximum Computer Guesses: . - A “button” labelled “Start Game”
- When this button is clicked, the games moves on to Step 2 below.
- Show 2 “input” fields on the screen where the user the user can tell the computer the “range” of numbers to choose from. For Example:
- Before displaying the main “game” area:
- The main “game” screen
- An output “span” on the screen indicating the current guess number.
- Example: Guess Number: 1
- An output “span” (where the computer indicates it’s guess of your number.
- Example: Are you thinking of the number: 50?
- Three Buttons where the user (player) will indicate “Higher” or “Lower” or “Correct”
- Notes:
- The computer should display a message (either directly on the screen or in the form of an alert box) indicating that the human must be “cheating” if the human responses are “impossible”.
- For example, if the user just keeps clicking “Higher”, meaning that the number would have to exceed 100!
- The computer should display a message (either directly on the screen or in the form of an alert box) if the maximum number of guesses has been exceeded. For Example:
Sorry, I could not guess your number within 5 guesses. - If guessed correctly, the computer should display a final message indicating how many guesses it took to correctly guess your number.
- The computer should display a message (either directly on the screen or in the form of an alert box) indicating that the human must be “cheating” if the human responses are “impossible”.
- An output “span” on the screen indicating the current guess number.
- The game should be broken up into two separate files, a .html and a .js file containing the HTML for the page and the JavaScript, respectively.
SUBMIT
- All HTML and JS files packaged into a single .ZIP file. (THIS IS IMPORTANT!)
HTML CODE:
<html> <head> <!-- The Code For the page --> <script src="Lab2.js"></script> </head> <body> <div id="DIV_SETUP"> <!-- Instructions / Setup --> <p>I'm Thinking of a number between <input ID="USER_LOW_VALUE" size="3"> and <input id="USER_HIGH_VALUE" size="3">. </p> <p> Maximum Number of Computer Guesses: <input ID="COMPUTER_MAX_GUESSES" size="3">. </p> <button id="START_GAME" onclick="startGame()">Start Game!</button> </div> <!-- NOTE: the "hidden" property can really be anything that is not "false", while setting hidden='true' works just in HTML5, hidden='hidden' is common for support of older browsers. --> <div id="DIV_PLAYAREA" hidden='hidden'> <!-- Game Play Area --> Guess Number: <span id="GUESS_NUM"></span> of <span id="GUESS_LIMIT"></span> <br> Are you thinking of the number: <span id="COMP_GUESS">50</span><br> <button id="BUTTON_HIGHER" onclick="handleUserChoice(this)">Higher</button> <button id="BUTTON_LOWER" onclick="handleUserChoice(this)">Lower</button> <button id="BUTTON_CORRECT" onclick="handleUserChoice(this)">Correct</button> </div> </body> </html>
JS CODE:
/* "OBJECT" to store all of the relevant game data. */ var gameData = { userMinRange: null, // The lower bound of initial range picked by the player userMaxRange: null, // The lower bound of initial range picked by the player maxGuessAllowed: null, // The Maximum number of guessed picked by the player. highestGuessSoFar: null, // The upper Bound of what the computer will choose lowestGuessSoFar: null, // The lower bound of what the computer will choose guessesSoFar: null, // The Number of guesses made by the computer so far. lastComputerGuess: null // The last guess made by the computer. }; /* NOTE YOU ARE FREE TO ADD ADDITIONAL FUNCTIONS WHERE NECESSARY */ /* Start the game */ function startGame() { // TODO: Populate the Object with the values from the DIV_SETUP. // TODO: Hide the SET_UP Area // TODO: Show the Play Area. // Update the game data values. gameData.userMinRange = get("USER_LOW_VALUE"); gameData.userMaxRange = get("USER_HIGH_VALUE"); gameData.maxGuessAllowed = get("COMPUTER_MAX_GUESSES"); gameData.guessesSoFar = 1; // Hide the setup area and show the play area document.getElementById("DIV_SETUP").hidden = true; document.getElementById("DIV_PLAYAREA").hidden = false; // Update the screen values shown to the user updatePlayArea(); // Just for debugging, so we can see the values as they change in the console. DEBUG_ShowGameData(); } /* Function (to be) called when game is finally over. * For example: Disable play buttons and display the number of guesses made. */ function endGame() { // TODO: Whatever should be displayed to the user upon game completion. // HINT: This could "disable" the user play buttons so they cannot be clicked anymore, // prompt the user to play again, etc. } /* Have the computer make a guess. */ function makeComputerGuess() { // TODO: Have the computer make a guess and update the last Guess made. // i.e., gameData.lastComputerGuess // // HINT: this function should date the highestGuessSoFar, lowestGuessSoFar, and lastComputerGuess } /* Update the Play Area with the new values. */ function updatePlayArea() { //TODO: Add all of the "set" functions to update the play area of the screen for all of the relevant elements. set("GUESS_NUM", gameData.guessesSoFar); set("GUESS_LIMIT", gameData.maxGuessAllowed); } /* Function to handle the users button click and call the other functions above as necessary. */ function handleUserChoice(theButton) { // TODO (potentially before *OR* after the "if" block): // - Respond to the users choice. // - Make next guess if necessary (at the appropriate time) // - End the game if the game should be over. // - Update the screen playarea at the appropriate time. // - Handle the number of guesses being >= the max allowed. if (theButton.id === "BUTTON_HIGHER") { // TODO: Handle Higher Button } else if (theButton.id === "BUTTON_LOWER") { // TODO: Handle Lower Button } else { // TODO: HANDLE CORRECT (What happens when the user clicks correct?) } } /* ****************************************************** */ /* ***** BELOW THIS POINT ARE SOME HELPER FUNCTIONS ***** */ /* FROM: * https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random * #Getting_a_random_integer_between_two_values_inclusive * */ function getRandomIntInclusive(min, max) { min = Math.ceil(min); max = Math.floor(max); //The maximum is inclusive and the minimum is inclusive var randomNumber = Math.floor(Math.random() * (max - min + 1)) + min; return randomNumber; } /* A Function to aid in debugging which just shows all of the gameData values. * NOTE: It can be removed once the development is complete. */ function DEBUG_ShowGameData() { for (var item in gameData) { // Test of ItemPropertyName = ItemPropertyValue var message = item + " = " + gameData[item]; console.log(message); } } /* Get the contents (value or innerHTML ) of an element on a page based it's ID. Throws an exception if the element ID does not exist. NOTE: The will usually be in "String" form. (numbers would need to be "parsed". */ function get(theObjectId) { // If the Id is invalid this could all fail, so be prepared if it does! try { // Get the page element var thePageElement = document.getElementById(theObjectId); // If the page element has a value, use it. if (thePageElement.value) { return thePageElement.value; } else { return thePageElement.innerHTML; } } catch (theError) { // A "Template Literal" where the value theObjectId is placed into the string. let errMessage = `Element "${theObjectId}" does not exist.n${theError.message}`; // Alert and log console.log(errMessage); alert(errMessage); // Throw a "new" error to prevent the program from continuing to run. throw errMessage; } } /* Same as above, but for setting... */ function set(theObjectId, theNewContents) { // If the Id is invalid this could all fail, so be prepared if it does! try { // Get the page element let thePageElement = document.getElementById(theObjectId); // If the page element has a value, use it. if (thePageElement.value) thePageElement.value = theNewContents; else thePageElement.innerHTML = theNewContents; } catch (theError) { // A "Template Literal" where the value theObjectId is placed into the string. let errMessage = `Element "${theObjectId}" does not exist.n${theError.message}`; // Alert and log console.log(errMessage); alert(errMessage); // Throw a "new" error to prevent the program from continuing to run. throw errMessage; } }
Looking for a solution written from scratch with No plagiarism and No AI?
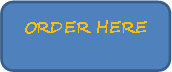
WHY CHOOSE US?
We deliver quality original papers |
Our experts write quality original papers using academic databases.We dont use AI in our work. We refund your money if AI is detected |
Free revisions |
We offer our clients multiple free revisions just to ensure you get what you want. |
Discounted prices |
All our prices are discounted which makes it affordable to you. Use code FIRST15 to get your discount |
100% originality |
We deliver papers that are written from scratch to deliver 100% originality. Our papers are free from plagiarism and NO similarity.We have ZERO TOLERANCE TO USE OF AI |
On-time delivery |
We will deliver your paper on time even on short notice or short deadline, overnight essay or even an urgent essay |